The Email feature enables Strapi applications to send emails from a server or an external provider.
Plan: Free feature.
Role & permission: Email > "send" permission for the user to send emails via the backend server.
Activation: Available by default.
Environment: Available in both Development & Production environment.
Configuration
Most configuration options for the Email feature are handled via your Strapi project's code. The Email feature is not configurable in the admin panel, however users can test email delivery if it has been setup by an administrator.
Admin panel settings
Path to configure the feature: Settings > Email feature > Configuration
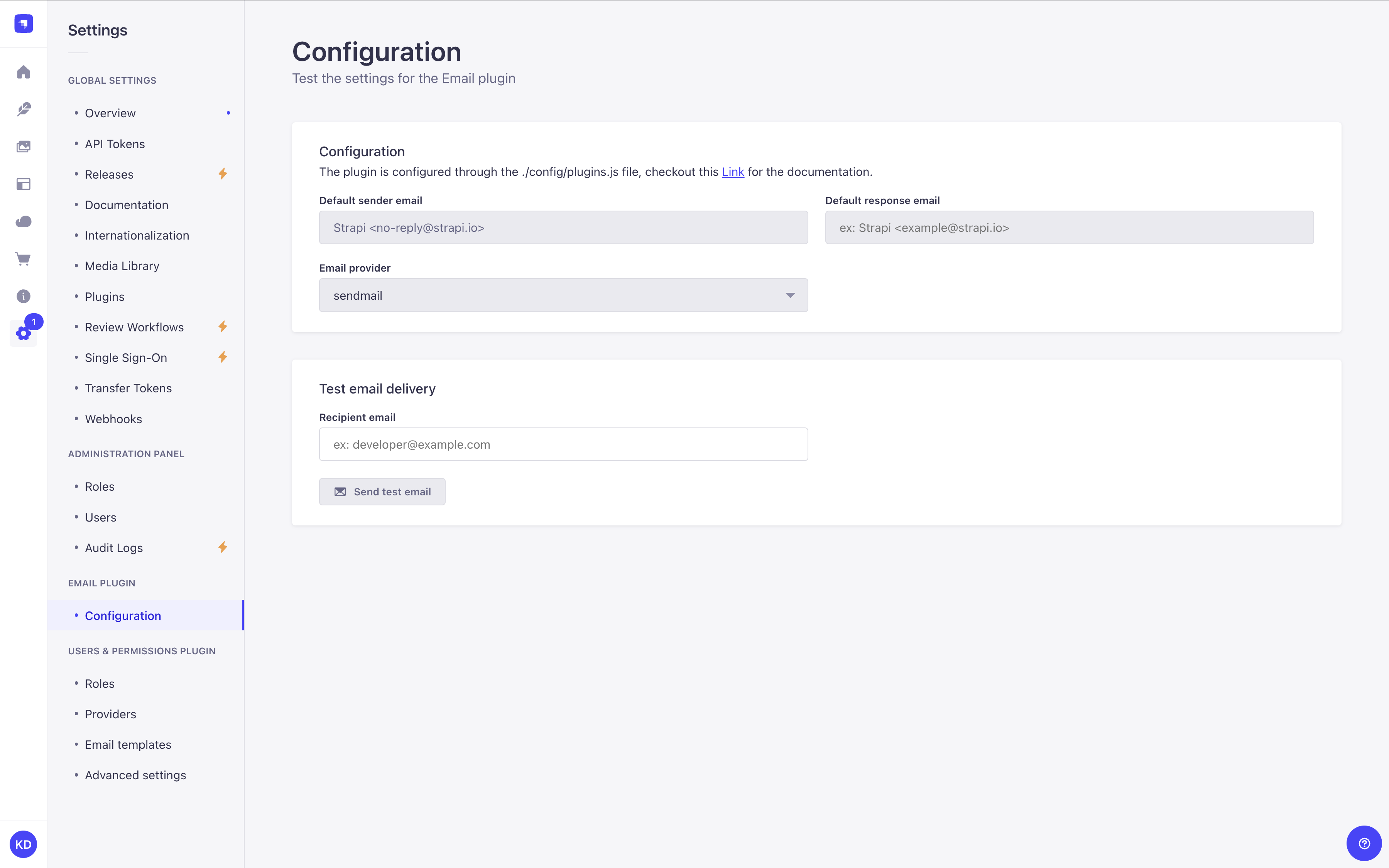
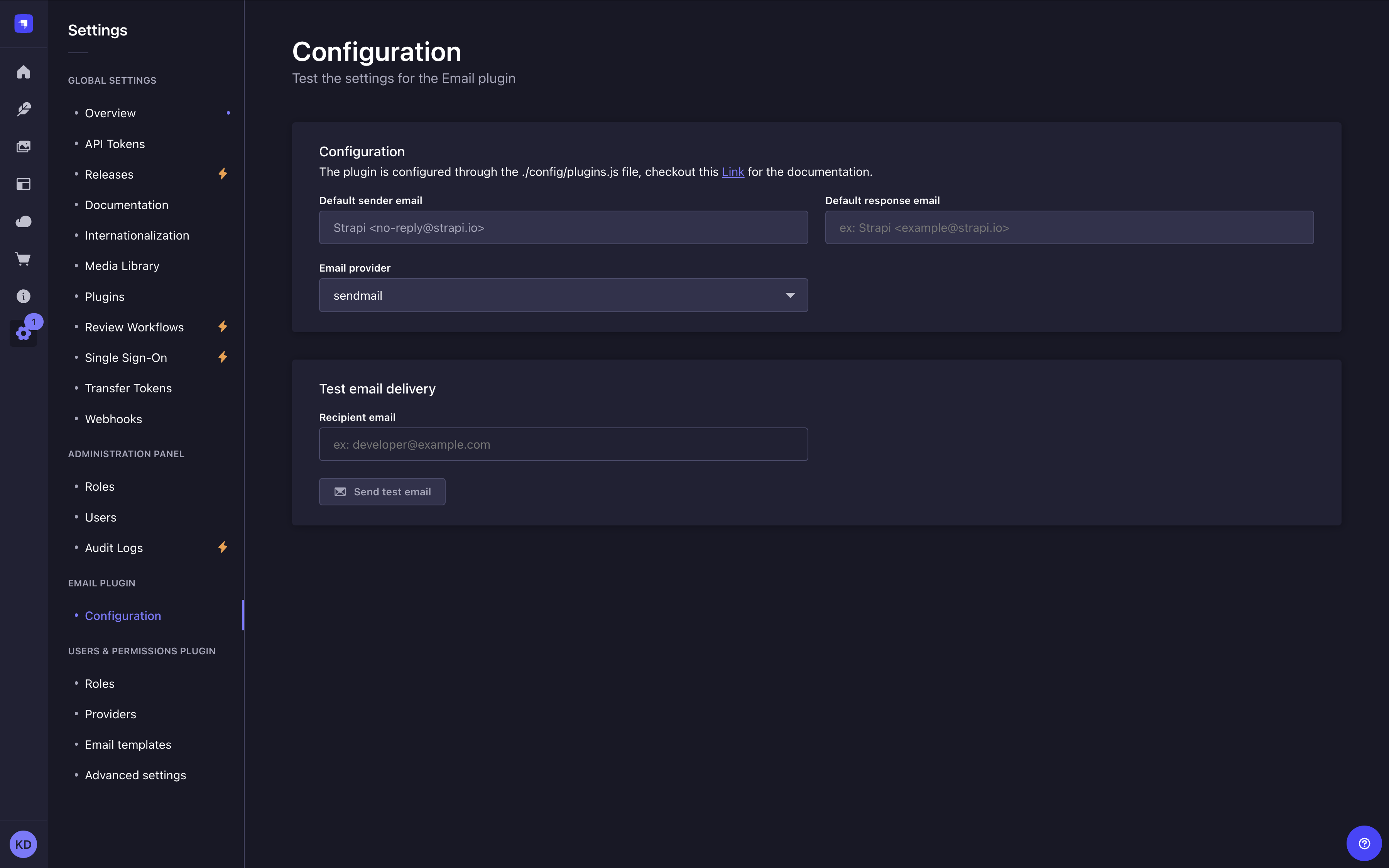
In the Configuration section, only the email address field under "Test email delivery" is modifiable by users. A send test email button sends a test email.
This page is only visible if the current role has the "Access the Email Settings page" permission enabled:
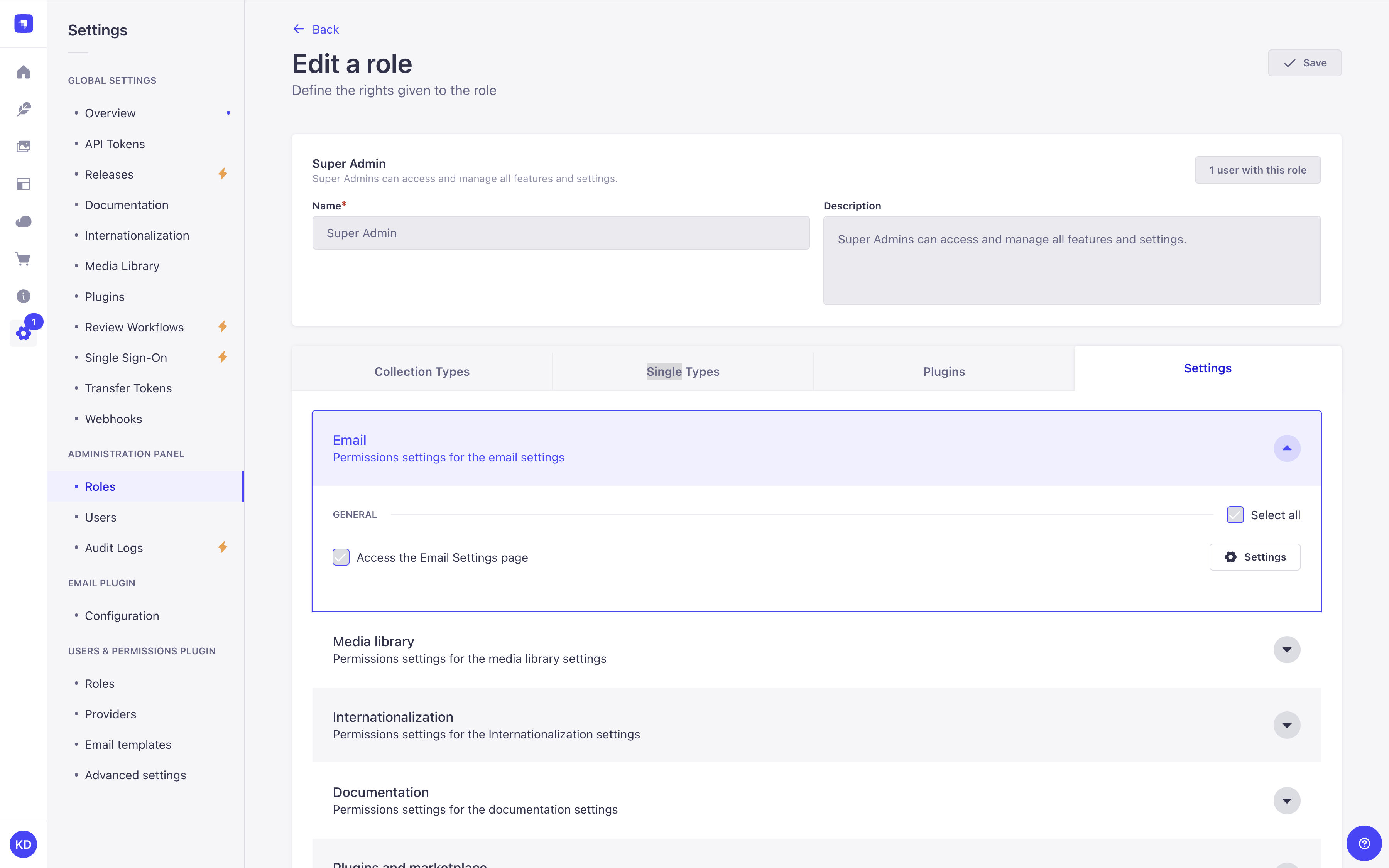
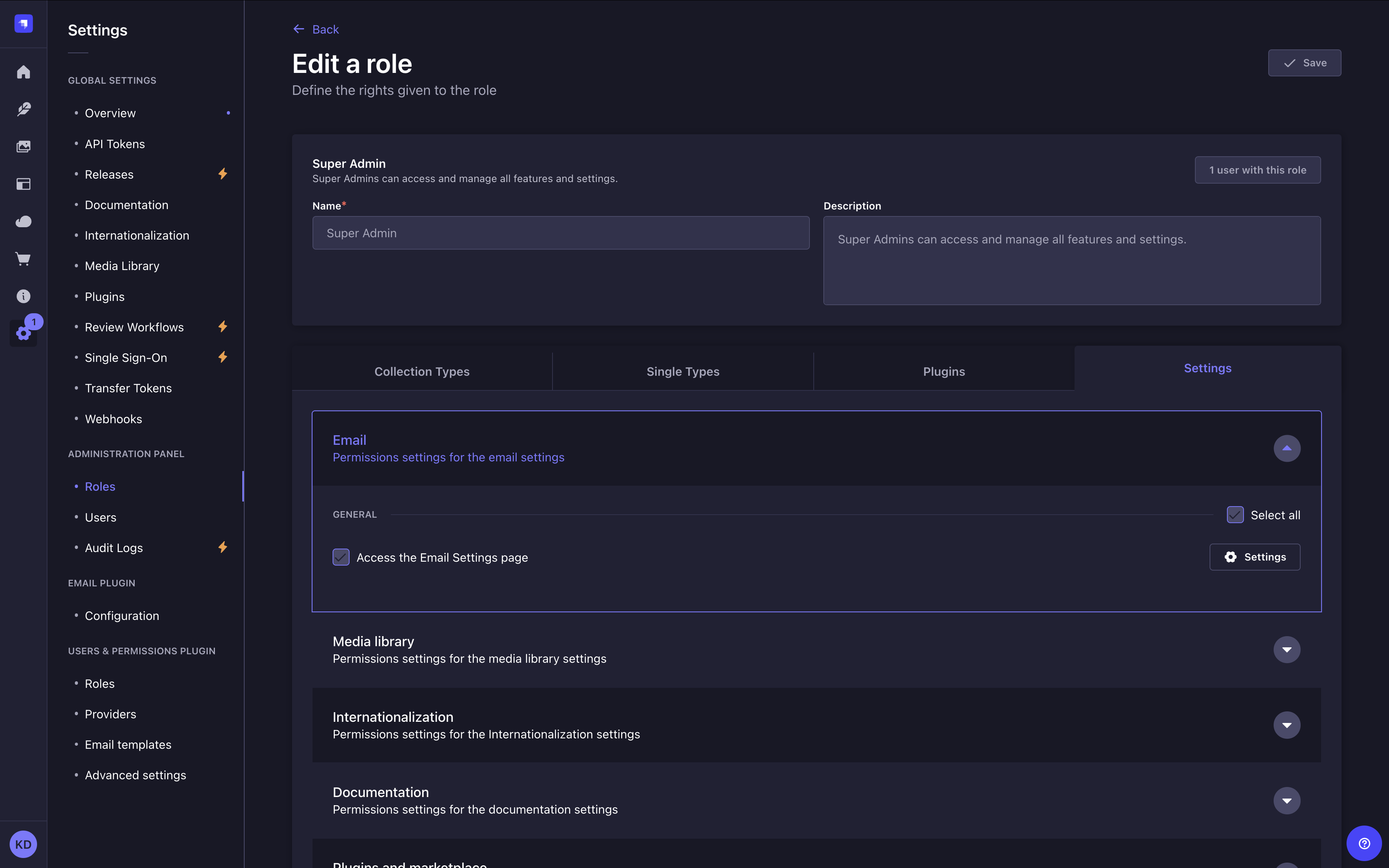
Code-based configuration
The Email feature requires a provider and a provider configuration in the config/plugins.js
file or config/plugins.ts
file. See the Providers documentation for detailed installation and configuration instructions.
Sendmail
is the default email provider in the Strapi Email feature. It provides functionality for the local development environment but is not production-ready in the default configuration. For production stage applications you need to further configure Sendmail
or change providers.
Usage
The Email feature uses the Strapi global API, meaning it can be called from anywhere inside a Strapi application, either from the back-end server itself through a controller or service, or from the admin panel, for example in response to an event (using lifecycle hooks).
Sending emails with a controller or service
The Email feature has an email
service that contains 2 functions to send emails:
send()
directly contains the email contents,sendTemplatedEmail()
consumes data from the Content Manager to populate emails, streamlining programmatic emails.
Using the send()
function
To trigger an email in response to a user action add the send()
function to a controller or service. The send function has the following properties:
Property | Type | Format | Description |
---|---|---|---|
from | string | email address | If not specified, uses defaultFrom in plugins.js . |
to | string | email address | Required |
cc | string | email address | Optional |
bcc | string | email address | Optional |
replyTo | string | email address | Optional |
subject | string | - | Required |
text | string | - | Either text or html is required. |
html | string | HTML | Either text or html is required. |
The following code example can be used in a controller or a service:
await strapi.plugins['email'].services.email.send({
to: 'valid email address',
from: 'your verified email address', //e.g. single sender verification in SendGrid
cc: 'valid email address',
bcc: 'valid email address',
replyTo: 'valid email address',
subject: 'The Strapi Email feature worked successfully',
text: 'Hello world!',
html: 'Hello world!',
}),
Using the sendTemplatedEmail()
function
The sendTemplatedEmail()
function is used to compose emails from a template. The function compiles the email from the available properties and then sends the email.
To use the sendTemplatedEmail()
function, define the emailTemplate
object and add the function to a controller or service. The function calls the emailTemplate
object, and can optionally call the emailOptions
and data
objects:
Parameter | Description | Type | Default |
---|---|---|---|
emailOptions Optional | Contains email addressing properties: to , from , replyTo , cc , and bcc | object | |
emailTemplate | Contains email content properties: subject , text , and html using Lodash string templates | object | |
data Optional | Contains the data used to compile the templates | object |
The following code example can be used in a controller or a service:
const emailTemplate = {
subject: 'Welcome <%= user.firstname %>',
text: `Welcome to mywebsite.fr!
Your account is now linked with: <%= user.email %>.`,
html: `<h1>Welcome to mywebsite.fr!</h1>
<p>Your account is now linked with: <%= user.email %>.<p>`,
};
await strapi.plugins['email'].services.email.sendTemplatedEmail(
{
to: user.email,
// from: is not specified, the defaultFrom is used.
},
emailTemplate,
{
user: _.pick(user, ['username', 'email', 'firstname', 'lastname']),
}
);
Sending emails from a lifecycle hook
To trigger an email based on administrator actions in the admin panel use lifecycle hooks and the send()
function.
The following example illustrates how to send an email each time a new content entry is added in the Content Manager use the afterCreate
lifecycle hook:
- JavaScript
- TypeScript
module.exports = {
async afterCreate(event) { // Connected to "Save" button in admin panel
const { result } = event;
try{
await strapi.plugin('email').service('email').send({ // you could also do: await strapi.service('plugin:email.email').send({
to: 'valid email address',
from: 'your verified email address', // e.g. single sender verification in SendGrid
cc: 'valid email address',
bcc: 'valid email address',
replyTo: 'valid email address',
subject: 'The Strapi Email feature worked successfully',
text: '${fieldName}', // Replace with a valid field ID
html: 'Hello world!',
})
} catch(err) {
console.log(err);
}
}
}
export default {
async afterCreate(event) { // Connected to "Save" button in admin panel
const { result } = event;
try{
await strapi.plugins['email'].services.email.send({
to: 'valid email address',
from: 'your verified email address', // e.g. single sender verification in SendGrid
cc: 'valid email address',
bcc: 'valid email address',
replyTo: 'valid email address',
subject: 'The Strapi Email feature worked successfully',
text: '${fieldName}', // Replace with a valid field ID
html: 'Hello world!',
})
} catch(err) {
console.log(err);
}
}
}